List_range_fill allows the user to take two distinct integer values in an array and create an object for each integer value in between even if they do not exist in the data payload.
Syntax
array list_range_fill(array $entry, int &keySelector, int &stepFunction, &constructor)
Takes an integer value (&keySelector
) within an array of objects ($entry
), creates missing objects in between existing values at an interval defined by &stepFunction
, and creates key/values within these object as defined by &constructor
.
Parameters
$entry: The input array.
&keySelector: The key in the input-array with missing entries that should be filled. Must be an integer.
&stepFunction: Sets the interval between each new object. Must be an integer.
&constructor: The definition for each newly created object. This can include three specific keys to allow for items in the original payload to be referenced:
__previous
: the previous item from the input list.__next
: the next item from the input list.__key
: the calculated value from using &stepFunction.
Example1
{
"input": [
{ "Name": "A", "Value": 2015 },
{ "Name": "B", "Value": 2017 },
{ "Name": "C", "Value": 2020 }
]
}
{
result: list_range_fill(input, &Value, &add(@,`1`), &{
Name:__previous.Name,
previousValue:__previous.Value,
nextValue:__next.Value,
Value: __key})
}
{
"result": [
{ "Name": "A", "Value": 2015 },
{ "Name": "A", "Value": 2016, "previousValue": 2015, "nextValue": 2017 },
{ "Name": "B", "Value": 2017 },
{ "Name": "B", "Value": 2018, "previousValue": 2017, "nextValue": 2020 },
{ "Name": "B", "Value": 2019, "previousValue": 2017, "nextValue": 2020 },
{ "Name": "C", "Value": 2020 }
]
}
This example lists each year between the first and last years of an array. It already has one year in between within the array. The previous and next years from the payload are also added to each object.
The &stepFunction in line one of the transformation is defined as &add(@,`1`)
. This ensures that we are adding 1 to the &Value
in each step to create our additional years. This is then referred to within our &constructor as Value: __key
.
Example 2
This function is also useful when creating a chart, where data points are missing. In the example below data from the year 2006, 2007, and 2009 are missing. This will make the chart look different compared to a data set where these data points are not missing. The function can change the chart on the left below to look like the chart to the right.
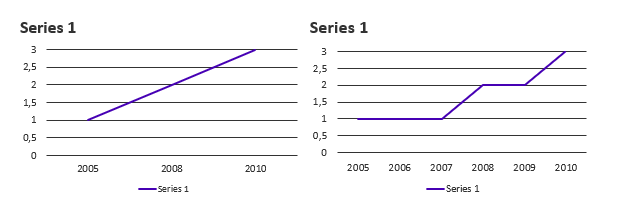
{
"values": [
{
"name": "a",
"year": 2005,
"value": 1
},
{
"name": "c",
"year": 2010,
"value": 3
},
{
"name": "b",
"year": 2008,
"value": 2
}
]
}
{
list_range_fill(
values,
&year,
&add(@, 1),
&{
last:__previous.name,
next:__next.name,
year: __key,
name:'?',
value: __previous.value
}
)
}
{
[
{
"name": "a",
"year": 2005,
"value": 1
},
{
"last": "a",
"next": "b",
"year": 2006,
"name": "?",
"value": 1
},
{
"last": "a",
"next": "b",
"year": 2007,
"name": "?",
"value": 1
},
{
"name": "b",
"year": 2008,
"value": 2
},
{
"last": "b",
"next": "c",
"year": 2009,
"name": "?",
"value": 2
},
{
"name": "c",
"year": 2010,
"value": 3
}
]
}
To ensure data points are added the function takes the list provided (values) and orders it by keySelector. The first key value is 2005, which the constructor finds in the values and returns without change. The function adds 1 to this key value to get 2006 which it does not find in values. The constructor creates a new object with each element created as defined. The function adds 1 to the previous key value to get 2007 which it does not find in values and creates another new object. The function adds 1 to the previous key value to get 2008 which it does find in the values and returns the data without change.
Notes
In building the list of new objects, the functionality determines whether or not an object already exists for it. If it does, it takes that entire object and does not build a new one. This can be seen in the example above. 2017 Already exists, so it is not recreated, hence it does not have a previousValue
or nextValue
defined.